수업소개
히든레이어와 멀티레이어의 구조를 이해하고, 히든레이어를 추가한 멀티레이어 인공신경망 모델을 완성해 봅니다.
강의
멀티레이어 신경망
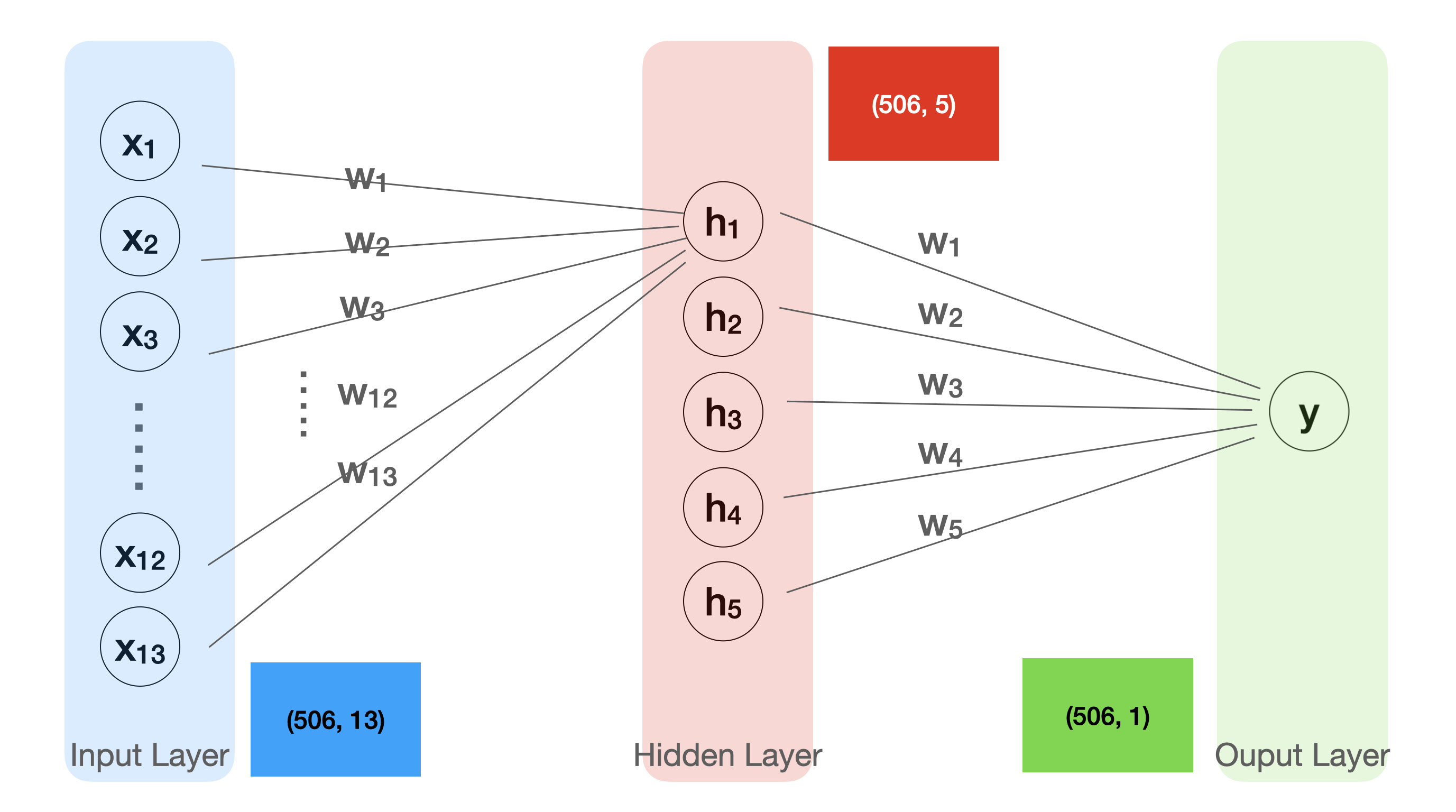
실습
소스코드
보스턴 집값 예측
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 | ########################## # 라이브러리 사용 import tensorflow as tf import pandas as pd ########################### # 1.과거의 데이터를 준비합니다. 보스턴 = pd.read_csv(파일경로) # 종속변수, 독립변수 독립 = 보스턴[[ 'crim' , 'zn' , 'indus' , 'chas' , 'nox' , 'rm' , 'age' , 'dis' , 'rad' , 'tax' , 'ptratio' , 'b' , 'lstat' ]] 종속 = 보스턴[[ 'medv' ]] print (독립.shape, 종속.shape) ########################### # 2. 모델의 구조를 만듭니다 X = tf.keras.layers. Input (shape = [ 13 ]) H = tf.keras.layers.Dense( 10 , activation = 'swish' )(X) Y = tf.keras.layers.Dense( 1 )(H) model = tf.keras.models.Model(X, Y) model. compile (loss = 'mse' ) # 모델 구조 확인 model.summary() ########################### # 3.데이터로 모델을 학습(FIT)합니다. model.fit(독립, 종속, epochs = 100 ) ########################### # 4. 모델을 이용합니다 print (model.predict(독립[: 5 ])) print (종속[: 5 ]) ########################### # 모델의 수식 확인 print (model.get_weights()) |
아이리스 품종 분류
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 | ########################### # 라이브러리 사용 import tensorflow as tf import pandas as pd ########################### # 1.과거의 데이터를 준비합니다. 아이리스 = pd.read_csv(파일경로) # 원핫인코딩 아이리스 = pd.get_dummies(아이리스) # 종속변수, 독립변수 독립 = 아이리스[[ '꽃잎길이' , '꽃잎폭' , '꽃받침길이' , '꽃받침폭' ]] 종속 = 아이리스[[ '품종_setosa' , '품종_versicolor' , '품종_virginica' ]] print (독립.shape, 종속.shape) ########################### # 2. 모델의 구조를 만듭니다 X = tf.keras.layers. Input (shape = [ 4 ]) H = tf.keras.layers.Dense( 8 , activation = "swish" )(X) H = tf.keras.layers.Dense( 8 , activation = "swish" )(H) H = tf.keras.layers.Dense( 8 , activation = "swish" )(H) Y = tf.keras.layers.Dense( 3 , activation = 'softmax' )(H) model = tf.keras.models.Model(X, Y) model. compile (loss = 'categorical_crossentropy' , metrics = 'accuracy' ) # 모델 구조 확인 model.summary() ########################### # 3.데이터로 모델을 학습(FIT)합니다. model.fit(독립, 종속, epochs = 100 ) ########################### # 4. 모델을 이용합니다 print (model.predict(독립[: 5 ])) print (종속[: 5 ]) |